International presence of indigenous artists#
This is a brief analysis of collected data from DAAO with a focus on international presence of indigenous artist/designers.
Import packages and pre-process data#
Show code cell source
import requests, gzip, io, os, json
# for data mgmt
import pandas as pd
import numpy as np
# for plotting
import matplotlib.pyplot as plt
import warnings
warnings.filterwarnings("ignore")
# provide folder_name which contains uncompressed data i.e., csv and jsonl files
# only need to change this if you have already downloaded data
# otherwise data will be fetched from google drive
global folder_name
folder_name = 'data/local'
def fetch_small_data_from_github(fname):
url = f"https://raw.githubusercontent.com/acd-engine/jupyterbook/master/data/analysis/{fname}"
response = requests.get(url)
rawdata = response.content.decode('utf-8')
return pd.read_csv(io.StringIO(rawdata))
def fetch_date_suffix():
url = f"https://raw.githubusercontent.com/acd-engine/jupyterbook/master/data/analysis/date_suffix"
response = requests.get(url)
rawdata = response.content.decode('utf-8')
try: return rawdata[:12]
except: return None
def check_if_csv_exists_in_folder(filename):
try: return pd.read_csv(os.path.join(folder_name, filename), low_memory=False)
except: return None
def fetch_data(filetype='csv', acdedata='organization'):
filename = f'acde_{acdedata}_{fetch_date_suffix()}.{filetype}'
# first check if the data exists in current directory
data_from_path = check_if_csv_exists_in_folder(filename)
if data_from_path is not None: return data_from_path
urls = fetch_small_data_from_github('acde_data_gdrive_urls.csv')
sharelink = urls[urls.data == acdedata][filetype].values[0]
url = f'https://drive.google.com/u/0/uc?id={sharelink}&export=download&confirm=yes'
response = requests.get(url)
decompressed_data = gzip.decompress(response.content)
decompressed_buffer = io.StringIO(decompressed_data.decode('utf-8'))
try:
if filetype == 'csv': df = pd.read_csv(decompressed_buffer, low_memory=False)
else: df = [json.loads(jl) for jl in pd.read_json(decompressed_buffer, lines=True, orient='records')[0]]
return pd.DataFrame(df)
except: return None
# uncomment if first time running; takes >1 mins to run
# persons = fetch_data(acdedata = 'person')
# events = fetch_data(acdedata = 'event')
We first list the most active indigenous artists/designers in terms of their international presence, followed by a time-series plot of distinct international exhibitions per year associated with indigenous artists/designers. This is followed by an interactive world map to highlight the relevant events. The last visualisation overlays the previous data alongside international activity by non-indigenous artists/designers.
Show code cell source
# # uncomment if first time running; takes 2 mins to run
# relevant_events = pd.DataFrame()
# for idx,row in persons[persons.is_indigenous == True].iterrows():
# try:
# this_person = pd.json_normalize(json.loads(row['related_events']))
# for event_id in this_person['object.ori_dbid.$oid'].values:
# this_event = events[events['ori_dbid'].str.contains(event_id, na=False)]['coverage_ranges'].values[0]
# this_event_df = pd.json_normalize(json.loads(this_event))
# this_event_df['name'] = row['display_name']
# this_event_df['event_id'] = event_id
# relevant_events = relevant_events.append(this_event_df)
# except: pass
# relevant_events.to_csv('data/local/DAAO_indigeneous_analysis_relevant_events.csv', index=False)
relevant_events = fetch_small_data_from_github('DAAO_indigeneous_analysis_relevant_events.csv')
# indigeneous artists/designers with the most international events
international_events = relevant_events[(relevant_events['place.address.country'] != 'Australia') &\
(relevant_events['place.address.country'].notnull())]
print('Indigenous artists/designers with the most international exhibitions:')
display(international_events['name'].value_counts().head(5))
years_raw = international_events[international_events['date_range.date_start.year'].notnull()]['date_range.date_start.year']\
.astype(int).to_list()
years = international_events[international_events['date_range.date_start.year'].notnull()]\
.drop_duplicates(subset=['event_id'])['date_range.date_start.year'].astype(int).to_list()
# plot a line plot of yearly count of international events over time
plt.figure(figsize=(10,5))
plt.plot(sorted(years), [years.count(y) for y in sorted(years)], marker='o', linewidth=0.5, markersize=4, alpha=0.8)
# set x-axis
plt.xticks(np.arange(1890, 2011, 10.0))
plt.xlabel('Year')
plt.ylabel('Count')
plt.title('Yearly count of distinct international exhibitions, Indigenous artists/designers (n=281)')
plt.show()
Indigenous artists/designers with the most international exhibitions:
"Jenny Fraser" 19
"Colin Tjakamarra McCormack" 15
"Gordon Bennett" 9
"Emily Kame Kngwarreye" 9
"Michael Riley" 8
Name: name, dtype: int64
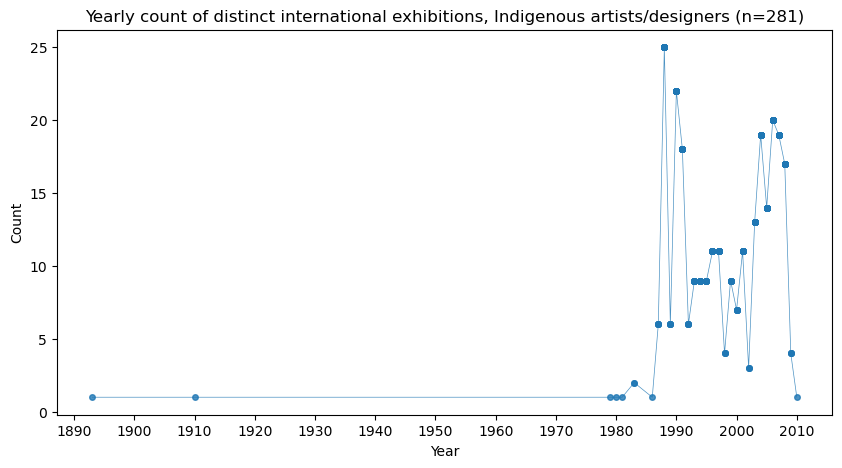
Show code cell source
# plot a light-weight world, point map of international events by
# place.geo_coord.latitude and place.geo_coord.longitude
import plotly.express as px
fig = px.scatter_geo(international_events[international_events['place.geo_coord.latitude'].notnull()],
lat='place.geo_coord.latitude', lon='place.geo_coord.longitude',\
color='place.address.country',
hover_data=['place.ori_address', 'date_range.date_start.year'],\
hover_name='name',\
projection='natural earth', opacity=0.5, size_max=10)
# add title
fig.update_layout(showlegend=False,
title_text='International exhibitions by indigenous artists/designers (n=284)')
# reduce figure wdith
fig.update_layout(width=800)
fig.show()
Show code cell source
# # uncomment if first time running; takes 12 mins to run
# relevant_events2 = pd.DataFrame()
# for idx,row in persons[persons.is_indigenous == False].iterrows():
# try:
# this_person = pd.json_normalize(json.loads(row['related_events']))
# for event_id in this_person['object.ori_dbid.$oid'].values:
# this_event = events[events['ori_dbid'].str.contains(event_id, na=False)]['coverage_ranges'].values[0]
# this_event_df = pd.json_normalize(json.loads(this_event))
# this_event_df['name'] = row['display_name']
# this_event_df['event_id'] = event_id
# relevant_events2 = relevant_events2.append(this_event_df)
# except: pass
# relevant_events2.to_csv('data/local/DAAO_indigeneous_analysis_relevant_events2.csv', index=False)
relevant_events2 = fetch_small_data_from_github('DAAO_indigeneous_analysis_relevant_events2.csv')
# indigenous artists/designers with the most international events
international_events2 = relevant_events2[(relevant_events2['place.address.country'] != 'Australia') &\
(relevant_events2['place.address.country'].notnull())]
print('Non-indigenous artists/designers with the most international events:')
display(international_events2['name'].value_counts().head(5))
years2_raw = international_events2[international_events2['date_range.date_start.year'].notnull()]['date_range.date_start.year']\
.astype(int).to_list()
# remove years before 1890 and after 2010
years2_raw = [y for y in years2_raw if y >= 1890 and y <= 2010]
years2 = international_events2[international_events2['date_range.date_start.year'].notnull()]\
.drop_duplicates(subset=['event_id'])['date_range.date_start.year'].astype(int).to_list()
# remove years before 1890 and after 2010
years2 = [y for y in years2 if y >= 1890 and y <= 2010]
# plot a line plot of yearly count of international events over time
plt.figure(figsize=(10,5))
plt.plot(sorted(years2), [years2.count(y) for y in sorted(years2)], label='Non-indigenous artists/designers',
marker='o', linewidth=0.5, markersize=4, color='orange', alpha=0.8)
plt.plot(sorted(years), [years.count(y) for y in sorted(years)], label='Indigenous artists/designers',
marker='o', linewidth=0.5, markersize=4, alpha=0.8)
plt.legend()
# set x-axis
plt.xticks(np.arange(1890, 2011, 10.0))
plt.xlabel('Year')
plt.ylabel('Count')
plt.title('Yearly count of international events')
plt.show()
Non-indigenous artists/designers with the most international events:
"Gwyn Hanssen Pigott" 66
"Mike Parr" 35
"James Fraser Scott" 33
"Stelarc" 27
"Luca Gansser" 27
Name: name, dtype: int64
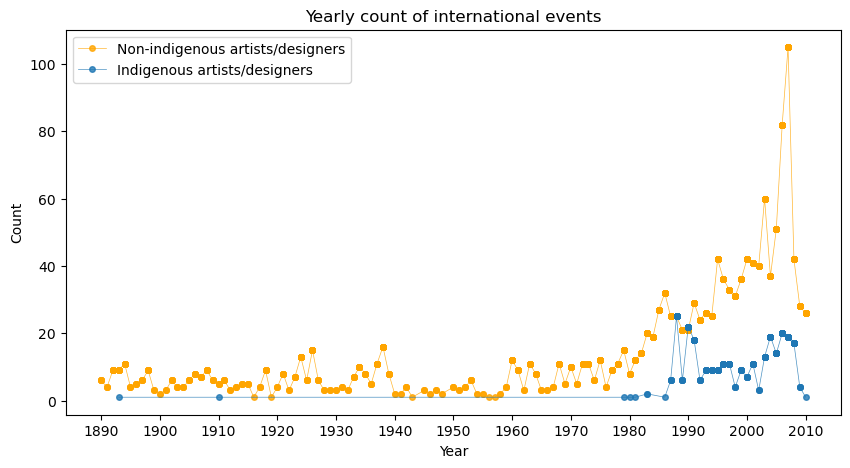
1893 in context
This year marks the first recorded entry of an indigeneous artist/designer participating in an international exhibition. According to the DAAO,
Mickey of Ulladulla was an Aboriginal artist active in the ninetenth century on the south coast of NSW. Five of his paintings were included posthumously in the catalogue of exhibits in the NSW Courts at the Chicago Exposition in 1893.
1910 in context
Another early entry occurred in 1910. According to the DAAO,
Celebrated shellworker, Emma Timbery of La Perouse, NSW, exhibited her shellworks in England in 1910.
1988 in context
The highest number of recorded exhibitions with indigenous artists/designers occurred in 1988. This is the only year where the number of international exhibitions with indigenous artists/designers (25) was equal to the number of international exhibitions with non-indigenous artists/designers (25).
16 out of these 25 exhibitions were held in the US. The rest were held in Tahiti (6), UK (2), and China (1).
Gauguin Museum in Tahiti held the most number of exhibitions in 1988 (6).
There are 21 distinct indigenous artists/designers:
Clifford Possum Tjapaltjarri exhibited three times (London, New York, and St Louis)
Colin Tjapanangka Dixon exhibited twice (Los Angeles and Tahiti)
Bessie Nakamarra Sims exhibited twice (Seattle and New York)
The rest exhibited once
1990 in context
This is the only year where the number of international exhibitions with indigenous artists/designers (22) exceeded the number of international exhibitions with non-indigenous artists/designers (21).
8 out of these 22 exhibitions were held in the US. The rest were held in the UK (7), France (5), Italy (3), Ireland (2), India (1), Vanuatu (1) and Spain (1).
Musée Fabre in Montepellier, France held the most number of exhibitions in 1990 (4).
There are 21 distinct indigenous artists/designers:
Gloria Petyarre exhibited three times (London, Dublin, and India)
Robert Campbell exhibited three times (Manchester, Montpellier, and Boston)
Nyukana Baker exhibited twice (Edinburgh and New Mexico)
Emily Kame Kngwarreye exhibited twice (Dublin and Boston)
Trevor Nickolls exhibited twice (Venice)
The rest exhibited once
Yearly count of international participations#
As opposed to distinct exhibitions, we also plot the number of people associated with each distinct exhibition. This allows us to inspect whether any networks exist at an international level.
Show code cell source
# plot a line plot of yearly count of international events over time
plt.figure(figsize=(10,5))
plt.plot(sorted(years2_raw), [years2_raw.count(y) for y in sorted(years2_raw)], label='Non-indigenous artists/designers',
marker='o', linewidth=0.5, markersize=4, color='orange', alpha=0.8)
plt.plot(sorted(years_raw), [years_raw.count(y) for y in sorted(years_raw)], label='Indigenous artists/designers',
marker='o', linewidth=0.5, markersize=4, alpha=0.8)
plt.legend()
# set x-axis
plt.xticks(np.arange(1890, 2011, 10.0))
plt.xlabel('Year')
plt.ylabel('Count')
plt.title('Yearly count of international participations')
plt.show()
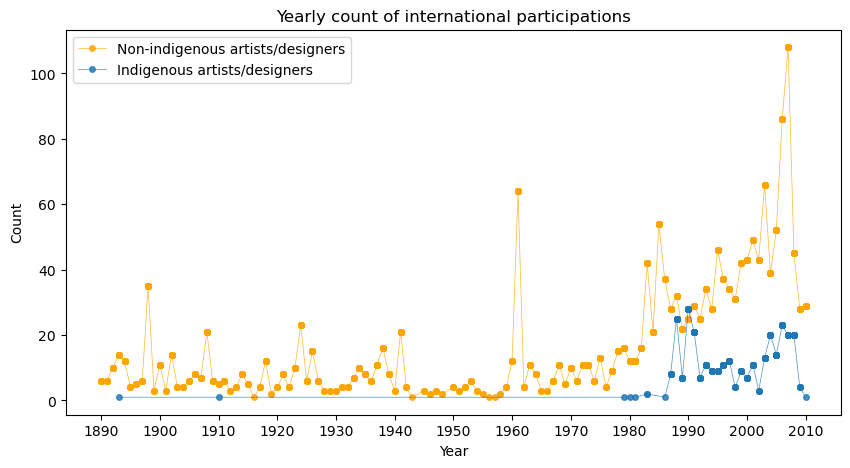
1898 in context
There are 35 non-indegenous artists/designers associated with international exhibitions in 1898. 27 of these artists/designers performed at the same exhibition in the UK. The exhibition was held at the Grafton Gallery in London. The exhibition was called Exhibition of Australian Art.
1961 in context
This peak consists of 64 non-indegenous artists/designers associated with international exhibitions in 1961. 55 of these artists/designers performed at the same exhibition in the UK. The exhibition was held at the Whitechapel Gallery in London between June and July. The exhibition was called Recent Australian Painting.
2007 in context
The highest peak occurred in 2007 which consisted of 108 non-indegenous artists/designers associated with international exhibitions. Most exhibitions are held across Europe. The exhibition network in 2007 is a lot more sparse than the aforementioned years with only two exhibitions including two distinct artists/designers from the DAAO. The rest of the exhibitions only include one artist/designer from the DAAO.
Key takeaways#
US (28%), UK (13%) and France (12%) are the top 3 countries with the highest number of exhibitions for indigenous artists/designers.
Similarly, UK (36%), US (13%) and France (10%) are also the top 3 countries with the highest number of exhibitions for non-indigenous artists/designers.
Considering raw counts, Greece is the only country that has more exhibitions for indigenous artists/designers than non-indigenous artists/designers.
In terms of proportion ratios,
Indigenous artists/designers are roughly three times more likely to have exhibitions in Austria and Netherlands
Indigenous artists/designers are roughly two times more likely to have exhibitions in US and Germany
France, Canada and China approximartely hold a one-to-one ratio of exhibitions for indigenous and non-indigenous artists/designers.
While Japan, New Zealand and UK are more likely to have exhibitions for non-indigenous artists/designers.
UK is almost three times more likely to have exhibitions for non-indigenous artists/designers than indigenous artists/designers.
Below we show countries with at least 5 exhibitions for indigenous artists/designers. This list is sorted by the ratio of exhibitions for indigenous artists/designers to non-indigenous artists/designers.
Show code cell source
from itables import show
indigenous_stats = pd.merge(international_events['place.address.country'].value_counts().reset_index(),
international_events['place.address.country'].value_counts(normalize=True).reset_index(), on='index').\
rename(columns={'index':'country', 'place.address.country_x':'count', 'place.address.country_y':'percentage'})
non_indigenous_stats = pd.merge(international_events2['place.address.country'].value_counts().reset_index(),
international_events2['place.address.country'].value_counts(normalize=True).reset_index(), on='index').\
rename(columns={'index':'country', 'place.address.country_x':'count', 'place.address.country_y':'percentage'})
all_stats = pd.merge(indigenous_stats,non_indigenous_stats, # how='outer',
on='country', suffixes=('_indigenous', '_non_indigenous'))
all_stats['ratio'] = all_stats['percentage_indigenous'] / all_stats['percentage_non_indigenous']
all_stats = all_stats[all_stats['count_indigenous'] > 5]
all_stats.sort_values('ratio', ascending=False)
# display data
show(all_stats.sort_values('ratio', ascending=False), scrollY="400px",
scrollCollapse=True, scrollX=True,
paging=False, showIndex=False, dom="tpr")
country | count_indigenous | percentage_indigenous | count_non_indigenous | percentage_non_indigenous | ratio |
---|---|---|---|---|---|
Loading... (need help?) |